The world of programming languages is vast and diverse, with each language offering unique features and capabilities. In this blog post, we will learn about two important features of C++ templates and exception handling. Understanding and mastering these concepts can significantly enhance your proficiency in C++ programming.
Templates and exception handling are pillars of C++ programming, providing solutions for generic programming and robust error management. Embracing these features unlocks the true potential of the language.
Template is a way of writing generic code that can work with different types of data. Exception handling is a way of dealing with errors or unexpected situations that may occur during the execution of a program.
Table of Contents
Concept of Template in C++
Template is one of the important features of C++ that allows for generic programming by creating functions and classes that work with any data type. They promote code reusability and enable the creation of flexible and efficient algorithms.
A template can be used to create a family of classes of functions. For example, a class template for an array class would enable us to create arrays of various data types such as int array and float array. Similarly, we can define a template for a function, say mul(), that would help us create various versions of mul() for multiplying int, float, and double type values.
A template can be considered as a macro which helps to create a family of classes or
functions. When an object of a specific type is defined for actual use, the template definition for that class is substituted with the required data type. Since a template is defined with a parameter that would be replaced by a specified data type at the time of actual use of the class or function, the templates are sometimes called parameterized classes or functions.
Advantages of Templates a) Easier to write b) Easier to understand c) They are typesafe
There are two types of templates:
- Function Templates
- Class Templates
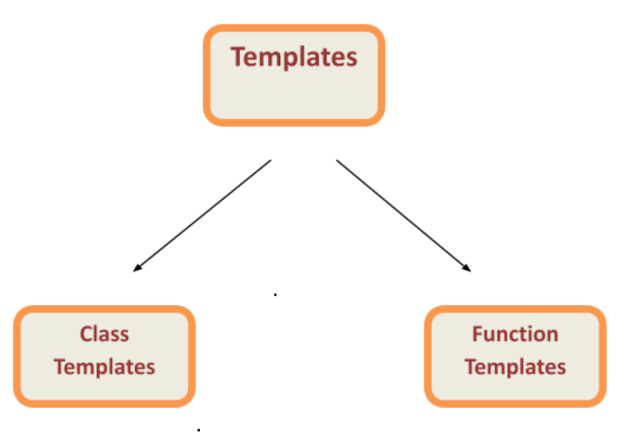
Function Template
Similar to the class templates, we can also define function templates that could be used to create a family of functions with different argument types. The general syntax of a function template is:
template <class T> return_type function_name (arguments of type T) { // - - - - // Body of function // with type T // wherever appropriate // - - - - }
The function template syntax is similar to that of the class template except that we are
defining functions instead of classes. We must use the template parameter T as and when necessary in the function body and its argument list.
The following example declares a swap() function template that will swap two values of a given type of data.
Example
#include <iostream.h>
template <class T>
void swap(T &x, T&y)
{
T temp=x ;
x=y ;
y=temp ;
}
void fun(int m, int n, float a, float b)
{ cout<< “m and n before swap:”<<m<< “ ”<<n<< “\n” ;
swap(m, n) ;
cout<< “m and n after swap:”<<m<< “ ”<<n<< “\n” ;
cout<< “a and b before swap:”<<a<< “ ”<<b<< “\n” ;
swap(a, b);
cout< “a and b after swap:”<<a<< “ ”<<b<< “\n” l
}
int main( )
{
fun(100, 200, 11.22, 33.44)
return 0 ;
}
Output:
m and n before swap : 100 200
m and n after swap : 200 100
Function overloading and problems
Overloading of Template Function
A template function may be overloaded either by template functions or ordinary functions of its name. In such cases, the overloading resolution is accomplished as follows:
- Call an ordinary function that has an exact match.
- Call a template function that could be created with an exact match.
- Try normal overloading resolution to ordinary functions and call the one that matches.
An error is generated if no match is found. Note that no automatic conversions are applied to arguments on the template functions. here is an example of how a template function is overloaded with an explicit function.
#include <iostream>
#include<string>
using namespace std;
template <class T>
void display(T x) {
cout<<"Template display: "<<x<<"\n";
}
void display(int x) { // overloads the generic display()
cout <<"Explicit display: "<<x<<"\n";
}
int main() {
display(100);
display(12.34);
display('c');
return 0;
}
Function templates with Multiple Parameters
Similar to template classes, we can use more than one generic data type in the template
statement using a comma-separated list as shown below:
template<class T1, class T2, ....> return_type function_name(arguments of types T1,T2, .....) { Body of function }
Example
#include <iostream.h>
template <class T1, class T2>
void show(T1x, T2 y)
{
cout<<x<< “ ”<<y<< “\n” ;
}
int main( )
{
show(1981, “C++”) ;
show(12.34, 1234) ;
return 0 ;
}
Output:
1981 C++
12.34 1234
Class Template
Class template is one of the kinds of templates that help us to create generic classes. It
is a simple process to create a generic class using a template with an anonymous type.
The general syntax of a class template is: template <class T> class name { // - - - // class member specification // with anonymous type T // wherever appropriate // - - - - - } ;
This syntax shows that the class template definition is very similar to an ordinary class
definition except for the use of prefix template & use of type T. These tell the compiler
that we are going to declare a template and use “T” as a type name in the declaration. This T can be replaced by any built-in data type (int, float, char, etc) or a user-defined data type.
Example
#include <iostream.h>
const size=3
template <class T>
class vector
{ T*V ; // type T vector
public:
vector( )
{ v=new T[size] ;
for (int i=0 ; i<size ; i++)
v[i]=a[i] ;
T operator*(vector &y)
{ T sum=0 ;
for (int i=0 ; i<size ; i++)
sum+=this→v[i]*y.v[i] ;
return sum ;
} } ;
int main( )
{ int x[3]={1, 2, 3} ;
int y[3]={4, 5, 6) ;
vector <int>v1 ;
vector<int>v2 ;
int R=v1*v2 ;
cout<< “R=”<<R<< “\n” ;
return 0 ;
}
Output
R=32
Example
#include <iostream.h>
template <class T>
class test
{ T a,b ;
public:
void getdata( )
{cin>>a>>b ;}
void putdata( )
{cout<< “You Entered:”<<a<< “ ”<<b ;}
} ;
int main( ) {
test<int>t1 ;
t1.getdata( ) ; t1.putdata( ) ;
test<float>t2 ;
t2.getdata( ) ; t2.putdata( ) ;
test(char>t3 ;
t3.getdata( ) ; t3.putdata( )
return 0 ;
}
Output
1 2
Derived class template
Class template with multiple parameter:
We can use more than one generic data type in the class template. They are declared as a comma separated list within the list specification as shown below:
template<class T1, class T2, .......> class classname { - - - - - - - - - - (Body of the class) - - - - - } ;
Example
#include <iostream.h>
template <class T1, class T2>
class Test
{ T1 a ;
T2 b ;
public:
Test(T1 x, T2 y)
{ a=x ;
b=y ;
}
void show( )
{
cout<<a<< “and” <<b<< “\n” ;
}
} ;
int main( )
{
Test<float, int> test1(1.54, 154) ;
Test<int, char> test2 (100, ‘w’) ;
test1.show( ) ;
test2.show( ) ;
return 0 ;
}
Output:
1.54 154
100 w
Concept of error handling
The most common types of error(also known as bugs) occur while programming in
C++ are Logic errors and Syntactic errors. The logic errors occur due to a poor understanding of the problem and solution procedure. The syntactic errors arise due to poor understanding of the language. These errors are detected by using exhaustive debugging and testing.
We often come across some peculiar problems other than logic or syntax errors. They
are known as exceptions. Exceptions are run-time anomalies or unusual conditions that a
program may encounter while executing. Anomalies might include conditions such as
division by zero, access to an array outside of its bounds, or running out of memory or disk space. ANSI C++ provides built-in language features to detect and handle exceptions which are basically run-time errors.
Exception handling was added to ANSII C++, providing a type_safe approach for coping with the unusual predictable problems that arise while executing a program. So, exception handling is the mechanism by using which we can identify and deal with such unusual conditions.
Basic of exception handling
Exceptions are of two kinds, namely, synchronous exceptions and asynchronous exceptions. Errors such as “out-of-range index” and “over-flow” belong to the synchronous type exceptions. The errors that are caused by events beyond the control of the program (such as keyboard interrupts) are called asynchronous exceptions. The proposed exception-handling mechanism in C++ is designed to handle only synchronous exceptions.
The purpose of the exception handling mechanism is to provide means to detect and report an “exceptional circumstance” so that appropriate action can be taken. The mechanism suggests a separate error-handling code that performs the following tasks:
- Find the problem (Hit the exception).
- Inform that an error has occurred (Throw the exception).
- Receive the error information (Catch the exception).
- Take corrective actions (Handle the exception).
The error handling code basically consists of two segments, one to detect errors and to throw exceptions, and the other to catch the exceptions and to take appropriate actions.
Exception handling mechanism: throw, catch and try
C++ exception handling mechanism is basically built upon three keywords, namely, try, throw, and catch. The keyword try is used to preface a block of statements (surrounded by braces) which may generate exceptions. This block of statements is known as try block. When an exception is detected, it is thrown using a throw statement in the try block. A catch block is defined by the keyword catch ‘catches’ and the exception ‘thrown’ by the throw statement in the try block, and handles it appropriately.
The catch block that catches an exception must immediately follow the try block that throws the exception. The general form of these two blocks are as follows:
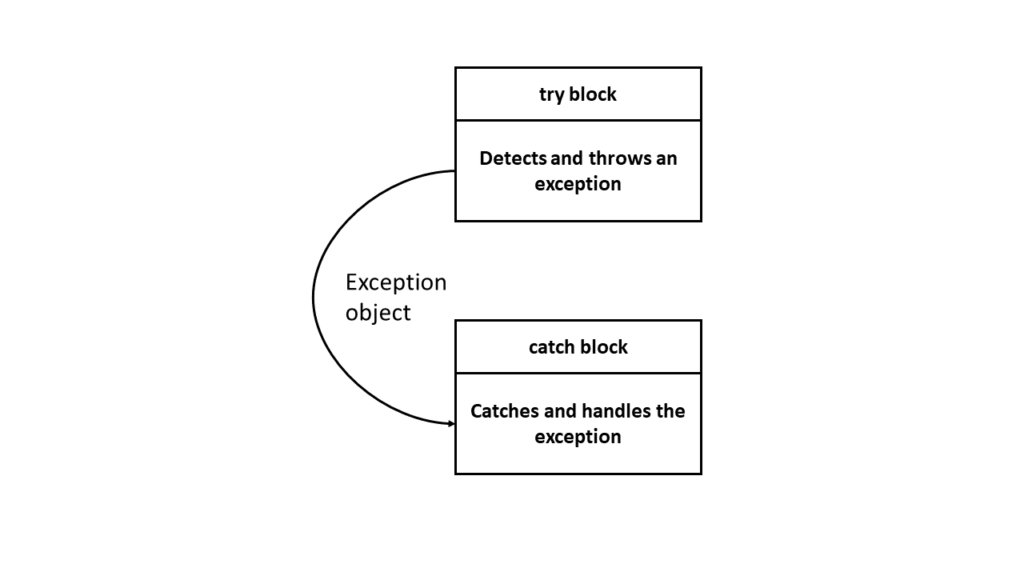
The general syntax is - - - - try { - - - - - - - - - // block of statement which detects and throws exception. throw exception ; - - - - } catch(type arg) { - - - - // block of statements that handles the exception - - - - }
When the try block throws an exception, the program control leaves the try block and enters the catch statement of the catch block. Note that exceptions are objects used to transmit information about a problem. If the type f object thrown matches the arg type in the catch statement, then catch block is executed for jandling the exception. If they do not match, the program is aborted with the help of abort( ) function which is invoked by default. When no execption is detected and thrown, the control goes to the statement immediately after the catch block. That is, the catch block is skipped.
Example of try-catch machanism
#include <iostream.h>
using namespace std;
int main() {
int a,b;
cout << " Enter values of a and b \n";
cin >> a;
cin >> b;
int x = a-b;
try
{
if(x != 0) {
cout << "Result(a/x) = " << a/x << "\n";
}
else {
throw(x);
}
}
catch(int i) {
cout << "Exception caught: x = " << x << "\n";
}
cout << "end";
return 0;
}
Output
First Run
Enter values of a and b
20 15
Result(a/x) = 4
end
Second Run
Enter values of a and b
10 10
Exception caught: x = 0
end
Exceptions are throw by functions that are invoked from within the try blocks. The point at which the throw is executed is called throw point. Once an exception is thrown to the catch block, control cannot return to the throw point.
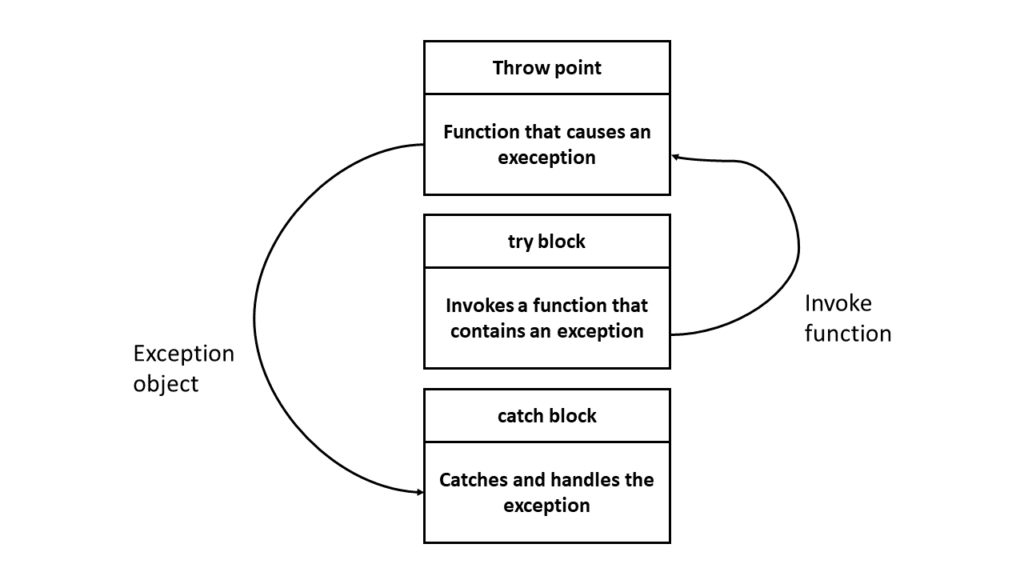
The general format of code for this kind of relationship is: type function(arg list) // function with exception { ............ ............ throw(object); // throws exception ............. } try { ............ ........... Invoke function here ............ } catch ( type arg) { ............. ............. handles exception here ............. } ................
FAQs
Are Templates Essential for Every C++ Project?
Templates are not mandatory for every project, but they offer significant benefits in terms of code flexibility and reusability. Consider using templates when you anticipate working with diverse data types or creating generic algorithms.
Can Exception Handling Replace Error Checks in C++?
While exception handling is a powerful mechanism for managing errors, it’s not a wholesale replacement for error checks. It’s best used for exceptional situations, allowing for graceful recovery, while error checks are crucial for routine error prevention.
Can Templates and Exception Handling Work Together?
Absolutely. Templates and exception handling complement each other. Exception handling ensures graceful error recovery, while templates enhance code flexibility. When used together judiciously, they contribute to creating robust and adaptable C++ code.
where to learn complete c++?
If you’re looking to learn C++, there are many resources available online. Here are some of the best ones: LearnCpp.com and Codecademy
Conclusion
Congratulations! You’ve traversed the intricacies of Template and Exception Handling In C++. Armed with this knowledge, you’re better equipped to navigate the challenges of C++ programming, creating resilient and efficient code. Embrace the power of templates and exception handling to elevate your coding prowess.