The most important feature of the Object and Class in C++. They form the backbone of object-oriented programming (OOP) and contribute significantly to code organization and reusability. Everything in C++ is associated with classes and objects. Along with its attributes and methods. For example: in real life, a car is an object. The car has attributes, such as weight and color, and methods, such as drive and brake.
Table of Contents
Concept of Object and Class In C++
An object in C++ is an instance of a class, A class in C++ is a user-defined data type that has data members and member functions. Data members are the variables that store the data of the object, and member functions are the functions that manipulate the data or perform some actions on the object. A class is like a blueprint for creating objects, and it defines the properties and behavior of the objects.
Specifying a Class in C++
A class is a way to bind the data and its associated function together. It allows the data (and functions) to be hidden, if necessary, from external use. When defining a class, we are creating a new abstract data type that can be treated like any other built-in data type. Generally, a class specification has two parts.
Class Declaration
The class declaration describes the type and scope of its members.
Class function definitions
The function definition describes how the class functions are implemented.
The general form of declaring a class is
class class_name { private: variable declarations ; function declarations ; public: variable declarations ; function declarations ; } ;
The class declaration is similar to a struct declaration. The keyword class specifies, that what follows is an abstract data of type class name. The body of a class is enclosed within braces and terminated by a semicolon. The class body contains the declaration of variables and functions. These functions and variables are collectively called class members. They are usually grouped under two sections, namely, private and public to denote which of the members are private and which of them are public. The keywords private and public are known as visibility labels. Note that these keywords are followed by a colon.
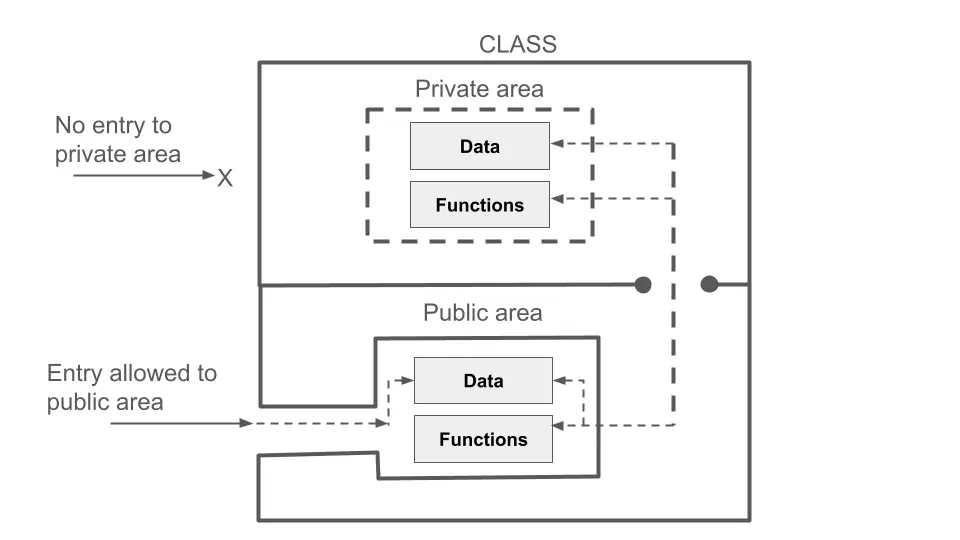
The class members that have been declared as private can be accessed only from within the class. On the other hand, public members can be accessed from outside the class also. The data hiding (using private declaration) is the key feature of object-oriented programming. The use of the keyword private is optional. By default, the members of a class are private. If both the labels are missing, then, by default, all the members are private. Such a class is completely hidden from the outside world and does not serve any purpose.
Define Data Member and Member Function
The variables declared inside the class are known as data members It may be private or public. the functions are known as member functions.
The private members cannot be accessed directly from outside of the class. The private
data of class can be accessed only by the member functions of that class. The public member can be accessed outside the class from the main function.
For Example class xyz { int x ; int y ; Public: int z ; } ; - - - - - - - - - - - - void main( ) { - - - - - - - - - - - - - - xyz p ; p.x=0 ; // error, x is private p.z=10 ; // ok, z is public - - - - - - - - - - - - }
The data member of a class is declared within the body of the class but member function can be defined in two ways
- Inside the class definition
- Outside the class definition
Inside the class definition
Normally, only small functions are defined inside the class definition. defining a member function is to replace the function declaration with the actual function definition inside the class.
When a function is defined inside a class, it is treated as an inline function. Therefore, all the restrictions and limitations that apply to an inline function are also applied here.
Syntax class class_name { public: return type member function (arg......) { // function body } } ;
Example 1
#include<iostream.h>
#include<conio.h>
class A {
int a,b;
public:
void getdata() {
Cin>>a>>b;
}
};
Example 2
#include<iostream.h>
#include<conio.h>
class item {
int number;
int cost;
public:
void getdata(int a, int b) {
number = a;
cost = b;
}
void display() {
Cout<<number=<<number<<"cost is"<<cost<<endl;
int total=number*cost;
Cout<<total cost="<<total<<endl;
}
};
void main() {
item x, y;
x.getdata(10,11);
x.displaydata();
y.getdata(10,8);
y.displaydata();
getch()
}
Outside the class definition
Member functions that are declared inside a class have to be defined separately outside the class. Their definitions are very much like normal functions. They should have a function header and a function body.
Syntax class class_name { // --------- public: return type member function(arg) //----------- } return type class_name :: member function(arg) // function body }
Example
#include <iostream.h>
#include<conio.h>
class ICTE {
private:
char name [20];
char college name [30];
int age;
public:
void getdata( );
};
void ICTE : : getdata() {
Cout<<"enter the name=";
Cin>>name;
Cout<<"enter the college name=";
Cin>>collegename;
Cout<<enter the age=";
Cin>>age;
}
void ICTE : : displaydata() {
Cout <<"name:"<<name<<endl;
Cout<<"collegename:"<<collegename<<endl;
Cout<<"age"<<endl;
}
void main() {
ICTE Student;
Student.getdata();
Student.displaydata();
getch();
}
Create object and access Member Function
Member functions are the functions, which have their declaration inside the class definition and works on the data members of the class. The definition of member functions can be inside or outside the definition of class.
If the member function is defined inside the class definition it can be defined directly, but if its defined outside the class, then we have to use the scope resolution : : operator along with class name along with function name.
The main function for both the function definition will be same. Inside main() we will create object of class, and will call the member function using dot . operator.
Syntax of accessing data member is: object_name . data_member
Syntax of accessing member function object_name . function_name
WAP to Create a class ICTE with data member name, college name, age and member function void getdata() and displaydata().
#include <iostream.h>
#include<conio.h>
class ICTE {
private:
char name [20];
char college name [30];
int age;
public:
void getdata( ) {
Cout<<"enter the name=";
Cin>>name;
Cout<<"enter the college name=";
Cin>>collegename;
Cout<<enter the age=";
Cin>>age;
}
void displaydata() {
Cout <<"name:"<<name<<endl;
Cout<<"collegename:"<<collegename<<endl;
Cout<<"age"<<endl;
}
}
WAP to Create a class ICTE with data member name and age function member, void getdata() and displaydata().
#include <iostream.h>
#include<conio.h>
class ICTE {
private:
char name [20];
int age;
public:
void getdata( ) {
Cout<<"enter the name=";
Cin>>name;
Cout<<enter the age=";
Cin>>age;
}
void displaydata() {
Cout <<"name:"<<name<<endl;
Cout<<"age"<<endl;
}
}
void main() {
ICTE Student;
Student.getdata();
Student.displaydata();
getch();
}
Making outer function inline
Inline function is a function that is expanded in line when it is called. When the inline function is called whole code of the inline function gets inserted or substituted at the point of inline function call. This substitution is performed by the C++ compiler at compile time. Inline function may increase efficiency if it is small.
The member function can call another member function within its definition which is called nesting of member function. It uses keyword ‘ inline ‘
The syntax for defining the function inline:
inline return-type function-name(parameters) { // function code }
class item { public: void getdata(int a, float b); // declaration }; inline void item :: getdata(int a, float b) // definition { number = a ; cost = b ; }
Example
#include <iostream>
using namespace std;
inline int cube(int s)
{
return s*s*s;
}
int main()
{
cout << "The cube of 3 is: " << cube(3) << "\n";
return 0;
}
//Output: The cube of 3 is: 27
Array with in Class
C++ provides the array, which stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. Array can be declared as the member of a class. arrays can be declared as private, public or protected members of class.
Declaring Arrays
To declare an array in C++, the programmer specifies the type of the elements and the number of elements required by an array as follows
type arrayName [ arraySize ];
Initializing Arrays
For Initializing C++ array elements either one by one or using a single statement.
double balance[] = {10.0, 24.0, 35.4, 1770.0, 560.0};
Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array
double salary = balance[4];
Example of Array as a class member
#include<iostream>
const int size=5;
class student
{
int roll_no;
int marks[size];
public:
void getdata ();
void tot_marks ();
} ;
void student :: getdata ()
{
cout<<"\nEnter roll no: ";
Cin>>roll_no;
for(int i=0; i<size; i++)
{
cout<<"Enter marks in subject"<<(i+1)<<": ";
cin>>marks[i] ;
}
void student :: tot_marks() { //calculating total marks
int total=0;
for(int i=0; i<size; i++)
total+ = marks[i];
cout<<"\n\nTotal marks "<<total;
}
void main()
student stu;
stu.getdata() ;
stu.tot_marks() ;
getch();
}
Array of Objects
Array can be created of any data type. Since a class is also a user defined data type, we can create array size of objects in object which is called an arrary of objects.
We know that an array can be of any data type including struct, Similarly, we can also have arrays of variables that are of the type class. Such variable are called arrays of objects. Consider the following class definition:
class employee { char name[30]; float age; public: void getdata(void); void putdata(void); };
The identifier employee is a user-defined data type and can be used to create ibhects that relate to different categories of the employees.
employee manage[3]; employee foreman[15]; employee worker[75];
Example for storing more than one Employee data.
#include<iostream> using namespace std; class Employee { int id; char name[30]; public: // Declaration of function void getdata(); void putdata(); }; // Defining the function outside the class void Employee::getdata() { cout << "Enter Id : "; cin >> id; cout << "Enter Name : "; cin >> name; } void Employee::putdata() { cout << id << " "; cout << name << " "; cout << endl; }
Static Data Member and Static Function
Static Data
Static data members are those data members which are declared by using a static keyword in front of the data member.
Properties
- It is initialized zero and only once when the first object of its class is created.
- Only one copy of that member is created for the entire class and is shared by all the objects of that class.
- It is accessible only within the class, but its lifetime is the entire program
Declaration
class abc { static int c ; - - - - - - - public: - - - - - - - - - - - - } ;
Example
#include<iostream.h>
#include<conio.h>
class student {
char name[20];
int roll;
static int count;
public:
void getdata() {
Cout<<"enter roll & name="<<endl;
Cin>>roll>>name;
count ++;
}
statuc void displaycount() {
Cout<<"Count:"<<Count<<endl;
}
};
int student :: count = 0;
void main () {
student a,b,c;
Student :: displaycount();
a.getdata();
b.getdata();
c.getdata();
getch();
}
Static Function
Static member functions are those that are declared by using the static in front of the member function and also we use static data members.
Properties
- A static function can access only other static member data and static member function declared in the same class.
- A static member function can be called using the same class name instead of its objects
Declaration
class abc { int n ; static int count ; public: - - - - - - - - - - static void output( ) { - - - - - - - - - - } } ;
Example
#include<iostream.h>
#include<conio.h>
class student {
char name[20];
int roll;
static int count;
public:
void getdata() {
Cout<<"enter roll & name="<<endl;
Cin>>roll>>name;
count ++;
}
statuc void displaycount() {
Cout<<"Count:"<<Count<<endl;
}
};
int student :: count = 0;
void main () {
student a,b,c;
Student :: displaycount();
a.getdata();
b.getdata();
c.getdata();
student :: display c;
getch();
}
Friends Functions
A friend function is a non-member function that has access to the private members of the class.
The outside functions can’t access the private data of a class. But there could be a
situation where we could like two classes to share a particular. C++ allows the common
function to have access to the private data of the class. Such a function may or may not be a member of any of the classes.
To make an outside function “friendly” to a class, we have to simply declare this function as a friend of the class as below.
class ABC { - - - - - - - - - - - - public : - - - - - - - - - - - - friend void xyz(void) ; //declaration } ; void xyz(void) //function definition { //function body }
The function declaration should be preceded by the keyword friend. The function definition does not use either the keyword friend or the scope operator ( : : ). The function that is declared with the keyword friend are known as friend functions. function can be declared as a friend in any number of classes.
Special Characteristics of Friend Function
- It is not in the scope of the class to which it has been declared as friend.
- That is why,it cannot be called using object of that class.
- It can be invoked like a normal function without the help of any object.
- It cannot access member names (member data) directly.
- It has to use an object name and dot membership operator with each member name.
- It can be declared in the public or the private part of a class without affecting its
meaning. - Usually, it has the objects as arguments.
Concept of Constructor and Destructor
Constructor
A constructor is a special member function that initializes the objects of its class. It is called special because its name is the same as that of the class name. It is called a constructor because it constructs the values of data members of the class.
A constructor is declared and defined as
// class with a constructor class cons { int data ; public: cons( ) ; // constructor declared - - - - - - - - - - - - } ; cons : : cons( ) // constructor defined { data=0 ; }
Characteristics of constructor
- Constructor has same name as that of its class name.
- It should be declared in public section of the class.
- It is invoked automatically when objects are created.
- It cannot return any value because it does not have a return type even void.
- It cannot have default arguments.
- It cannot be a virtual function and we cannot refer to its address.
- It cannot be inherited.
- It makes implicit call to operators new and delete when memory allocation is
required.
Example
#include <iostream.h>
#include <conio.h>
class sample
{
int a, b ;
public:
sample( )
{
cout<< “This is constructor” << endl ;
a=150; b=350 ;
}
int add( )
{
return(a+b) ;
}
} ;
void main( )
{
clrscr( ) ;
sample s ; //constructor called
cout<< “Sum of a & b value is:”<<s.add( )<<endl ;
getch( ) ;
}
Destructor
The complement of a constructor is the destructor. Destructors are the special function that destroys the object that has been created by a constructor. The destructor is called automatically by the compiler when the object goes out of scope to clean up storage taken by objects. The objects are destroyed in the reverse order from their creation in the constructor. Destructors too have a special name a class name preceded by a tilde sign ( ~ ).
Syntax Class A { public: ~ A ( ); };
Example 1 #include <iostream.h> class myclass { int a ; public: myclass( ) ; // constructor ~ myclass( ) ; // destructor void show( ) ; } ; myclass: : myclass( ) { cout<< “\n constructor \n” ; a=10 ; } myclass: :~ myclass( ) { cout<< “Destructing .... \n” ; } Example 2 #include<iostream.h> #include<conio.h> class example { private: int data*; public: ~ example() { Cout<<"inside the ICTE class we learn"; } example() { Cout<<"ICTE class destroy"; } }; void main() { example e; getch(); }
Types Of Constructor
Default / Empty Constructor
It is possible to define a constructor with default argument like in normal function. A constructor that takes no argument is called default / empty constructor.
Syntax class abc { .................. public: { abc() } };
Example #include<iostream.h> #include<conio.h> class Sukuna { public: Sukuna() { Cout<<"We are Students"; } }; void main() { Sukuna students; getch(); };
Parameterized constructor
A constructor that takes an argument is called a parameterized constructor.
It is possible to pass one or more arguments to a constructor function. Simply add the
appropriate parameters to the constructor function’s declaration and definition. Then, when you declare an object, specify the arguments.
Syntax class abc { public: abc (int data) };
Example #include<iostream.h> #include<conio.h> class student { int roll; public: student (int x) { roll = x; } void display() { Cout<<roll; } }; void main() { student s1(7) s1.display(); getch(); }
Copy constructor
The constructor having reference parameter is called copy constructor. Copy constrcutors are used to copy one object to another one.
Declaration for copy constructor
class abc { int x, y ; public: abc (abc &c1) { x=c1.x ; y=c1.y ; } abc( ) { x=10 ; y=20 ; } } ;
Example #include<iostream.h> #include<conio.h> class student { int roll; public: student (int x) { roll = x; } student (student & y) { roll = y.roll; } void display() { Cout<<roll; } }; void main() { student s1(7); s1.display(); student s2(5); s2.display(); getch(); }
Example of using two class #including <iostream> using namespace std; class code { int id; public: code() { } // constructor code(int a) { // constructor again id = a; } code(code & x){ // copy constructor id = x.id; // copy in the value } void display(void) { cout<<id; } } int main() { code A(100); code B(A); code C = A; code D; D = A; cout << "\n id of A: "; A.display(); cout << "\n id of B: "; B.display(); cout << "\n id of C: "; C.display(); cout << "\n id of D: "; D.display(); return 0; }
FAQs
What is the difference between an object and a class in C++?
An object is an instance of a class that encapsulates data and behavior, while a class acts as a blueprint defining the structure and behavior of objects.
How do constructors differ from destructors in C++?
Constructors initialize objects when they’re created, whereas destructors clean up resources when objects go out of scope or are explicitly destroyed.
Where to go for Further Understanding?
To deepen your understanding of objects and classes in C++, here are some valuable resources Tutorialspoint and Cplusplus.com
Conclusion
Mastering the concepts of classes and objects in C++ is fundamental for proficient programming. This comprehensive guide has provided insights into their definitions, implementation, and relationship, enabling developers to create robust and scalable code structures.