Inheritance In C++ is the most powerful feature of OOPs. Inheritance is the process of creating a new class(derived class) from the existing class(base class).
Table of Contents
Concept of Inheritance
Inheritance is the most powerful feature of object-oriented programming after classes
and objects. Inheritance is the process of creating a new class, called derived class from
existing class, called base class. The derived class inherits some or all the traits from base
class. The base class is unchanged by this. The most important advantage of inheritance is reusability. Once a base class is written and debugged, it need not be touched again and we can use this class for deriving another class if we need. Reusing existing code saves time and money. By reusability, a programmer can use a class created by another person or company and without modifying it derive other class from it.
Base and Derived Class
In the process of inheritance, the existing classes that are used to derive new classes are called base classes and the new classes derived from the existing class are called derived classes.
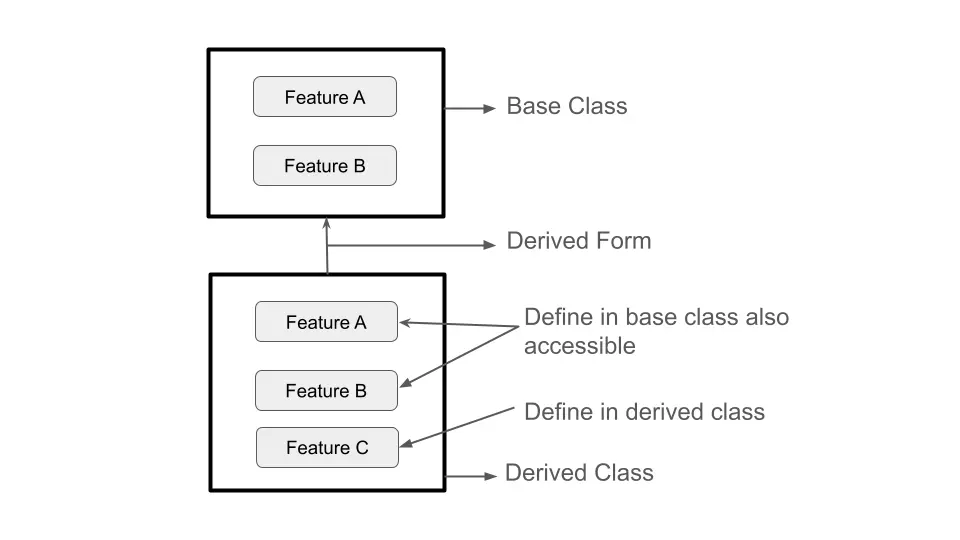
Private, Public, and Protected Specifier
Visibility Modifier (Access Specifier):
Visibility Modifier (Access Specifier) | Accessible from own class | Accessible from derived class | Accessible from objects outside class |
---|---|---|---|
public | yes | yes | yes |
private | yes | no | no |
protected | yes | yes | no |
Base Class Visibility | Derived Class Visibility | ||
---|---|---|---|
Public derivation | Private derivation | Protected derivation | |
Private | Not inherited | Not inherited | Not inherited |
Protected | Protected | Private | Protected |
Public | Public | Private | Protected |
Derived class declaration
A derived class can be defined by specifying its relationship with the base class in
addition to its own detail.
The general syntax is class derived_class_name : visibility_mode base_class_name { ....... //members of derived class } ;
where, the colon (;) indicates that the derived_class_name is derived from the base_class_name. The visibility_mode is optional, if present, may be either private or public. The default visibility mode is private. Visibility mode specifies whether the features of the base class are privately derived or publicly derived or derived on protected.
Examples: class ABC : private XYZ // private derivation { members of ABC } ; class ABC : public XYZ // public derivation { members of ABC } ; class ABC : protected XYZ // protected derivation { members of ABC } ; class ABC : XYZ // private derivation by default { members of ABC } ;
While any derived_class is inherited from a base_class, following things should be
understood
- When a base class is privately inherited by a derived class, only the public and
protected members of the base class can be accessed by the member functions of the derived class. This means no private member of the base class can be accessed by the objects of the derived class. Public and protected member of the base class becomes private in the derived class. - When a base class is publicly inherited by a derived class the private members are not inherited, the public and protected are inherited. The public members of base class becomes public in derived class where as protected members of base class becomes protected in derived class.
- When a base class is protectly inherited by a derived class, then public members of
base class becomes protected in derived class ; protected members of base class
becomes protected in the derived class, the private members of the base class are not inherited by the derived class but note that we can access private members through
inherited member function of the base class.
Member function overriding
In C++, function overriding is a feature that allows a derived class to provide a specific implementation of a function that is already provided by its base class1. The function that is redefined in the derived class is said to override the function in the base class.
Here are some key points about function overriding in C++:
- Same Function Name: The function in the derived class should have the same name as the function in the base class.
- Same Parameter List: The function in the derived class must have the same parameters as the function in the base class
- Different Function Body: The function in the derived class has a different function body than the function in the base class
Types and Levels of Inheritance in C++
Inheritance are classified into following types
Single Inheritance
When a class is derived from only one base class, then it is called single Inheritance.
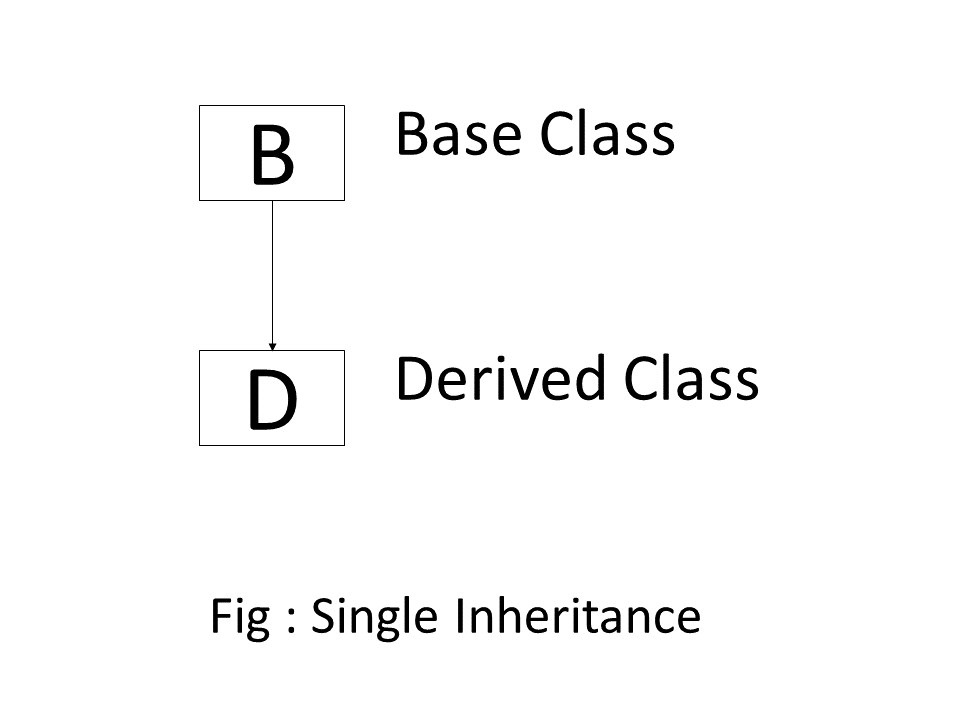
It is represented as B is derived from class A Class A { { Class B:A { };
Example Program
#include <iostream.h>
#include<conio.h>
class A {
public:
int x;
};
class B : public A {
int y, sum;
public:
void getdata() {
cout<<"enter x=";
cin>>x;
cout<<"enter y=";
cin>>y;
sum=x+y;
cout<<sum;
}
};
void main() {
B b1;
b1.getdata();
getch();
}
Multiple Inheritance
If a class is derived from more than one base class then inheritance is called as multiple inheritance. Multiple inheritance allows us to combine the features of several existing classes as a starting point for defining new class. The derivation is private, public or protected. Note this is also possible that one derivation is public and another one is protected or private, etc
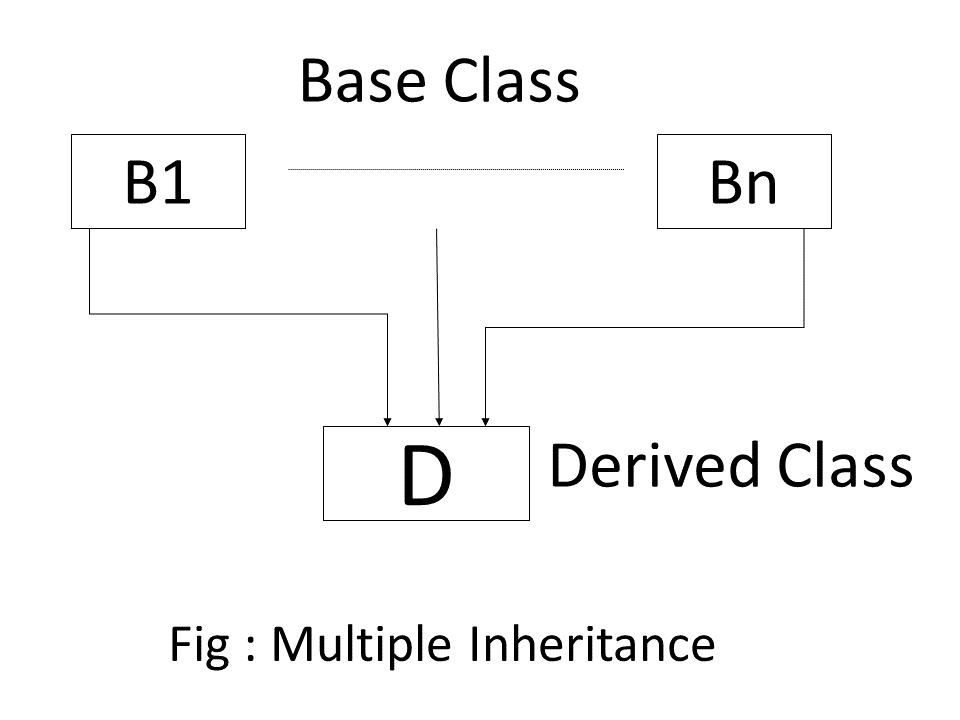
The syntax of multiple inheritance is: class D: derivation B1, derivation B2 ........ { member of class D } ;
Example
#include <iostream.h>
#include <conio.h>
class biodata { char name[20] ;
char semester[20] ;
int age ;
int rn ;
public: void getbiodata( ) ;
void showbiodata( ) ;
} ;
class marks { char sub[10] ;
float total ;
public:
void getrm( ) ;
void showm( ) ; } ;
class final: public biodata, public marks
{ char fteacher[20] ;
public: void getf( ) ;
void showf( ) ; } ;
void biodata: : getbiodata( )
{
cout<< “Enter name:” ; cin>>name ;
cout<< “Enter semester:” ; cin>>semester ;
cout<< “Enter age:” ; cin>>age ;
cout<< “Enter rn:” ; cin>>rn ; }
void biodata: : showbiodata( )
{
cout<< “Name:”<<name<<endl ;
cout<< “Semester:”<<semester<<endl ;
cout<< “Age:”<<age<<endl ;
cout<< “Rn:”<<rn<<endl ;
void marks: : getm( )
{
cout<< “Enter subject name:” ; cin>>sub ;
cout<< “Enter marks:” ; cin>>total ; }
void marks: : showm( )
{
cout<< “Subject name:”<<sub<<endl ;
cout<< “Marks are:”<<total<<endl ; }
void final: : getf( )
{ cout<< “Enter your favourite teacher” ; cin>>teacher ; }
void final: : showf( )
{ cout<< “Favourite teacher:”<<fteacehr<<endl ; }
void main( )
{ final f ; clrscr( ) ;
f.getbiodata( ) ;
f.getm( ) ;
f.getf( ) ;
f.showbiodata( ) ;
f.shown( ) ;
f.showf( ) ;
getch( ) ;
}
Output:
Enter name : archana
Enter semester : six
Enter age : 20
Enter rn : 10
Enter subject name : C++
Enter marks : 85
Enter favourite teacher : Ram
Name : archana
Semester : six
Age : 20
Rn : 10
Subject name : C++
Marks are : 85
Favourite teacher : Ram
Multilevel Inheritance
The mechanism of deriving a class from another derived class is called multilevel. A derived class with multilevel inheritance is declared as follows:
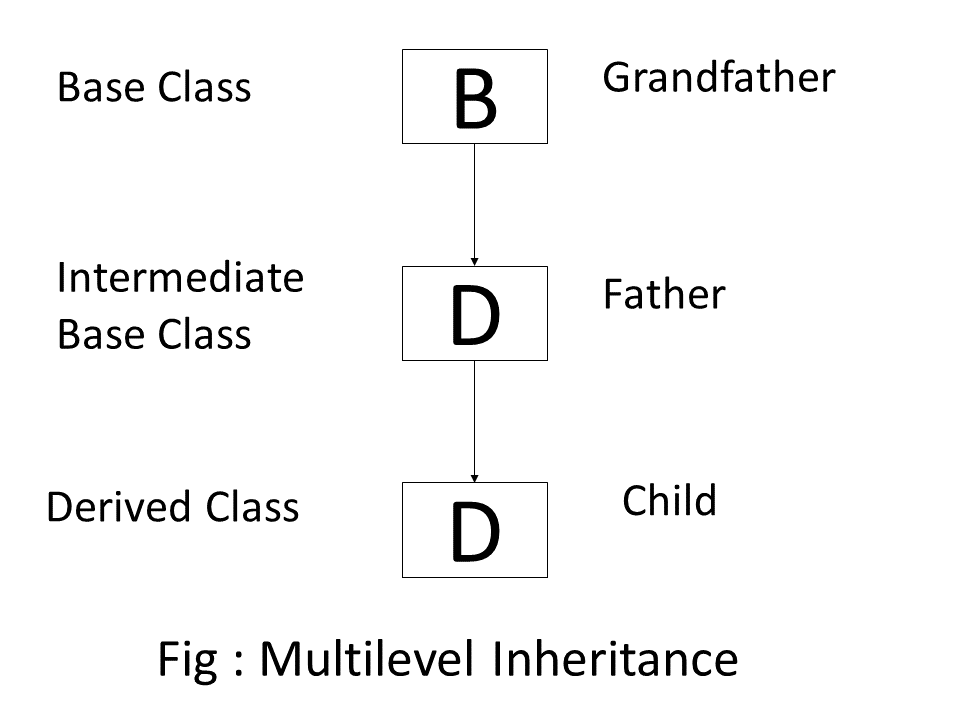
class A{...........}; // Base class class B: public A {...........}; // B derived from A class C: public B {...........}; // C derived from B // This process can be extended to any number of levels.
Example
#include <iostream.h>
class std
{
protected : char name[20] ;
int rn ;
public : void.getdata( )
{
cout<< “Student=” ; cin>>name ;
cout<< “Roll no.=” ; cin>>rn ; }
void showdata( )
{
cout<< “Student=”<<name<<endl ;
cout<< “Roll no=”<<rn<<endl ;
} ; // end of class std
class marks : public std {
protected : int m1, m2 ;
public:
void getm( )
{
cout<< “enter marks in Maths:”
cin>>m1 ;
cout<< “enter marks in English=” ; cin>>m2 ;
}
void showm( ) {
cout<< “Maths”<<m1<<endl ; cout<< “English=”<<m2<<endl ;
}
} ; //end of class marks
class result : public marks
{
int total ;
public: void calculate( )
{ total=m1+m2 ; }
void show( )
{
cout<< “Total marks=”<<total ; }
} ; //end of class result
void main( )
{
result s1 ;
s1.getdata( ) ;
s1.getm( ) ;
s1.calculate( ) ;
s1.showdata( ) ;
s1.shown( ) ;
s1.show( ) ;
}
Output
Student=ram
Roll no=4
Enter marks in maths=56
Enter marks in english=45
Student=ram
Roll no=4
Maths=56
English=45
Total marks=101
Hierarchical Inheritance
When two or more classes are derived from one base class, it is called hierarchical Inheritance.
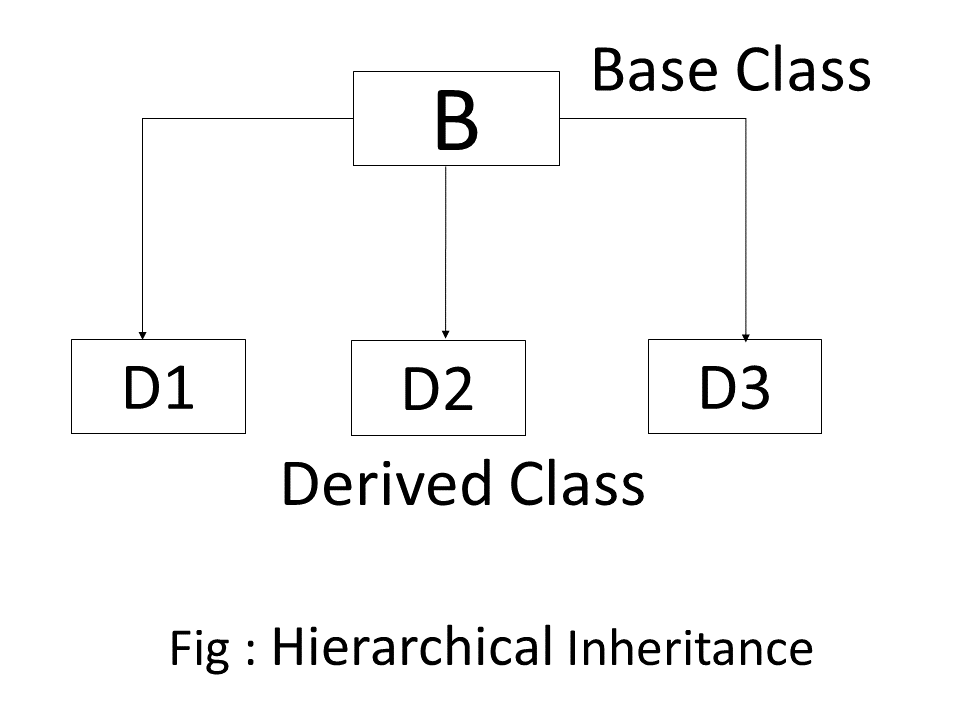
Syntax class A { ......... ......... }; class B : public A { ......... ......... }; class C : public A { ......... ......... }; class D : public A
// Example
#include<iostream.h>
#include<conio.h>
class base {
protected:
int x,y;
public:
void getdata() {
cout<<" enter X and Y" <<endl;
cin>>x>>y;
}
};
class derived : public base {
public:
void sum() {
int s;
s= x+y;
cout<<"Sum=Sum"s<<endl;
}
};
class derived d1: public base {
public :
void diff() {
int diff;
diff = x-y;
cout<<diff="<<diff<<endl;
}
};
void main() {
derive d;
derive d1;
d.getdata();
d.sum();
d1.getdata();
d1.diff();
getch();
}
Hybrid Inheritance
This inheritance is the combination of multiple and hierarchical inheritance.
In other words, If we apply more than one type of inheritance to design a problem then that is known as
hybrid inheritance.
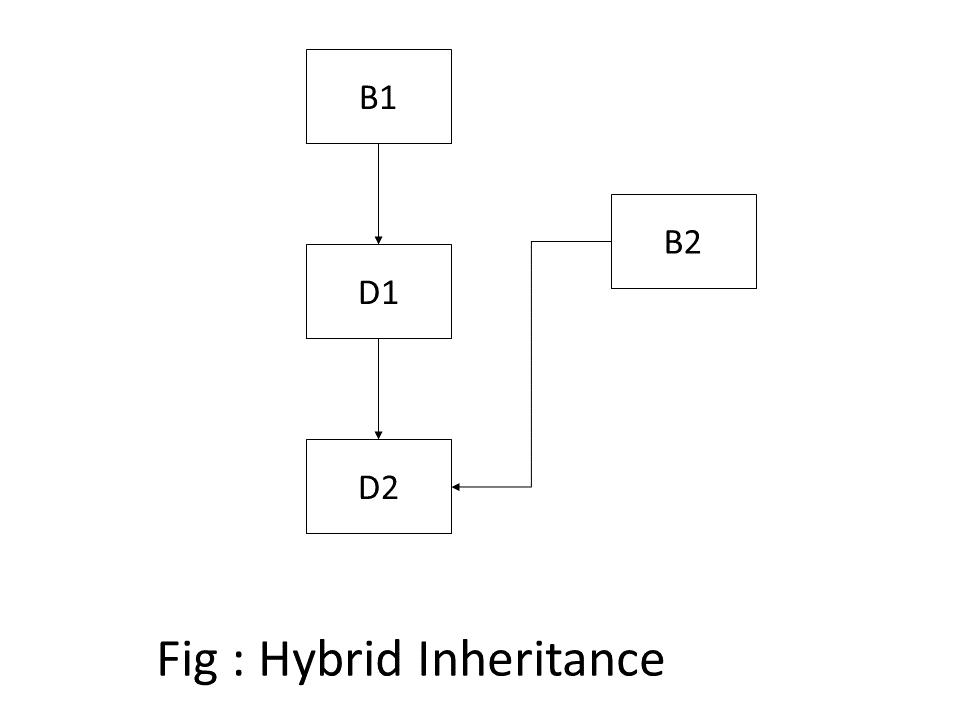
Example
#include<iostream.h>
#include<conio.h>
class arithmetic {
protected:
int num1, num2;
public:
void getdata() {
cout<<"for Addition";
cout<<"enter the first number";
cin>>num1;
cout<<"enter the second number";
cin>>num2;
}
};
class plus : public arithmetic {
protected
int sum;
public: void add() {
sum = num1 + num2;
}
};
class minus {
protected:
int n1,n2,diff;
public: void sub() {
cout<<"for subtraction:";
cout<<"enter the first number";
cin>>n1;
cout<<"enter the second number";
cin>>n2;
diff = n1-n2;
}
};
class result : public plus, public minus {
public : void display() {
cout<<"Sum of"<<num1<<"and"<<num2<<"="<<sum;
cout<<"Difference of "<<n1<<"and"<<n2<<"="<<diff;
}
};
void main() {
result z;
z.getdata();
z.add();
z.sub();
z.display();
getch();
}
Output
For Addition:
enter the first number = 10
enter the SECOND number = 5
For Subtraction:
enter the first number = 10
enter the SECOND number = 5
Sum of 10 and 5 = 15
Difference of 10 and 5 = 5
Ambiguity problems in inheritance
We may face a problem in using multiple inheritance when a function with the same inheritance appears in more than one base class which is.
Class A { Public: void display() { cout<<"Class A"; } }; Class B { public: void display() { cout<<"Class B"; } };
Which displays() function is used by the derived class when we inherit these two classes? We can solve this problem by defining a name with the function as follows:
Class C : public A, public B { public: void display() { A : : display() } }; Now, we can use derived class as follows void main() { C c1; c1.display(); getch(); }
Constructor in Derived Class
The constructor of the derived class works for it. base class, such constructors are called constructors in the derived class or common contructors.
Using Constructors and Destructors in Inheritance
It is possible for the base class, the derived class or both to have a constructor and/or
destructor. When a base class and a derived class both have constructor and destructor functions, the constructor functions are executed in order of derivation. The destructor functions are executed in reverse order. That is the base class constructor is executed before the constructor in the derived class. The reverse is true for destructor functions: the destructor in the derived class is executed before the base class destructor.
So far, we have passed arguments to either the derived class or the base class constructor. When only the derived class takes an initialization, arguments are passed to the derived class constructor in the normal fashion.
However, if we need to pass an argument to the constructor of the base class, a little more effort is needed:
- All necessary arguments to both the class and derived class are passed to the derived class constructor.
- Using an expanded form of the derived class’ constructor declaration, we then pass
the appropriate arguments along with the base class
The general form of defining a derived constructor is:
derived_constructor (arg_list) : base(arg_list) { body of the derived class constructor }
Here, base is the name of the base class. It is permissible for both the derived class and
the base class to use the same argument. The derived class can ignore all
arguments and just pass them along to the base.
// Illustrate when base and derived class constructor and destructor functions are executed
#include <iostream.h>
class base {
public:
base( ) { cout<< “Constructing base \n” ; }
base( ) {cout<< “Destructing base \n” ; }
} ;
class derived : public base {
public:
derived( ) { cout<< “Constructing derived \n” ; }
~ derived( ) { cout<< “Destructing derived \n” ; }
} ;
int main( )
derived obj ;
return 0 ;
}
Output:
Constructing base
Constructing derived
Destructing derived
Destructing base
// Program shows how to pass argument to both base class and derived class
#include <iostream.h>
class base {
int i ;
public :
base (int n) {
cout<< “Constructing base \n” ;
i=n ; }
~ base( ) { cout<< “Destructing base \n” ; }
void showi( ) { cout<<i<< “\n” ; }
} ;
class derived: public base {int j ;
public:
derived (int n): base(n) // pass argument to the base class
{ cout<< “Constructing derived \n” ;
j=n ; }
~ derived( ) {cout<< “Destructing derived \n”;}
void show( ) { cout<<j<< “\n” ; }
} ;
void main( ) {derived o(10) ;
o.showi( ) o.showj( ) ; }
Output:
Constructing base
Constructing derived
10
10
Destructing derived
Destructing base
Extending operator overloading in the derived class
In C++, operator overloading allows you to redefine the way operators work for user-defined types. When it comes to inheritance, there are some important points to consider
- Inheritance of Overloaded Operators: All overloaded operators except assignment (operator=) are inherited by derived classes.
- Overloading in Derived Class: If a derived class defines an operator that is already present in the base class, the operator in the base class will be hidden. This is because the compiler, when it finds an operator in the derived class, does not look for other overloads of the operator in the base class
- No Overload Resolution Between Base and Derived: Overloading doesn’t work for derived classes in C++. There is no overload resolution between Base and Derived4. If a derived class and its base class both have overloaded operators, the compiler will not be able to resolve which one to use based on the context.
- Extending Overloading in Derived Class: If you want to extend the functionality of an overloaded operator from a base class in a derived class, you would need to explicitly call the base class’s operator inside the derived class’s operator.
Benefits and Cost of Inheritance
The benefits of inheritance are listed below:
- It supports the concept of hierarchical classification.
- The derived class inherits some or all the properties of the base class.
- Inheritance provides the concept of reusability. This means an additional feature can be added to an existing class without modifying the original class. This helps to save
development time and reduce the cost of maintenance. - Code sharing can occur at several places.
- It will permit the construction of reusable software components. Already such
libraries are commercially available. - The new software system can be generated more quickly and conveniently by rapid
prototyping. - Programmers can divide their work themselves and later on combine their codes.
- The base and derived classes get tightly coupled. This means one cannot be used
independently of the other that is to say they are interconnected to each other. - We know that inheritance uses the concept of reusability of program codes so the
defects in the original code module might be transferred to the new module thereby resulting in defective modules.
Conclusion
Inheritance is a powerful feature in C++ that promotes code reusability and can make your code more organized and easier to manage. However, it should be used wisely, as improper use of inheritance can lead to problems in your code.
FAQs
What is inheritance in C++?
Inheritance allows a class to inherit properties and behaviors from another class, fostering code reuse.
How does inheritance promote code reuse?
By enabling the creation of new classes based on existing ones, reducing redundancy and promoting modularity.
What are the access specifiers in C++ inheritance?
Access specifiers (public, private, protected) determine the accessibility of inherited members in derived classes.
Can multiple inheritance cause ambiguity?
Yes, multiple inheritance can lead to ambiguity when two base classes have a member with the same name.
Where I can learn about Inheritance?
you can also explore Tutorialspoint for further learning